Hiya!
I'm trying to give the animals in my game a state in which they wander around a pasture, but despite the state and target_position seemingly updating correctly, the sprite doesn't move around.
Here's a screenshot with the output, showing that the target_position does update:
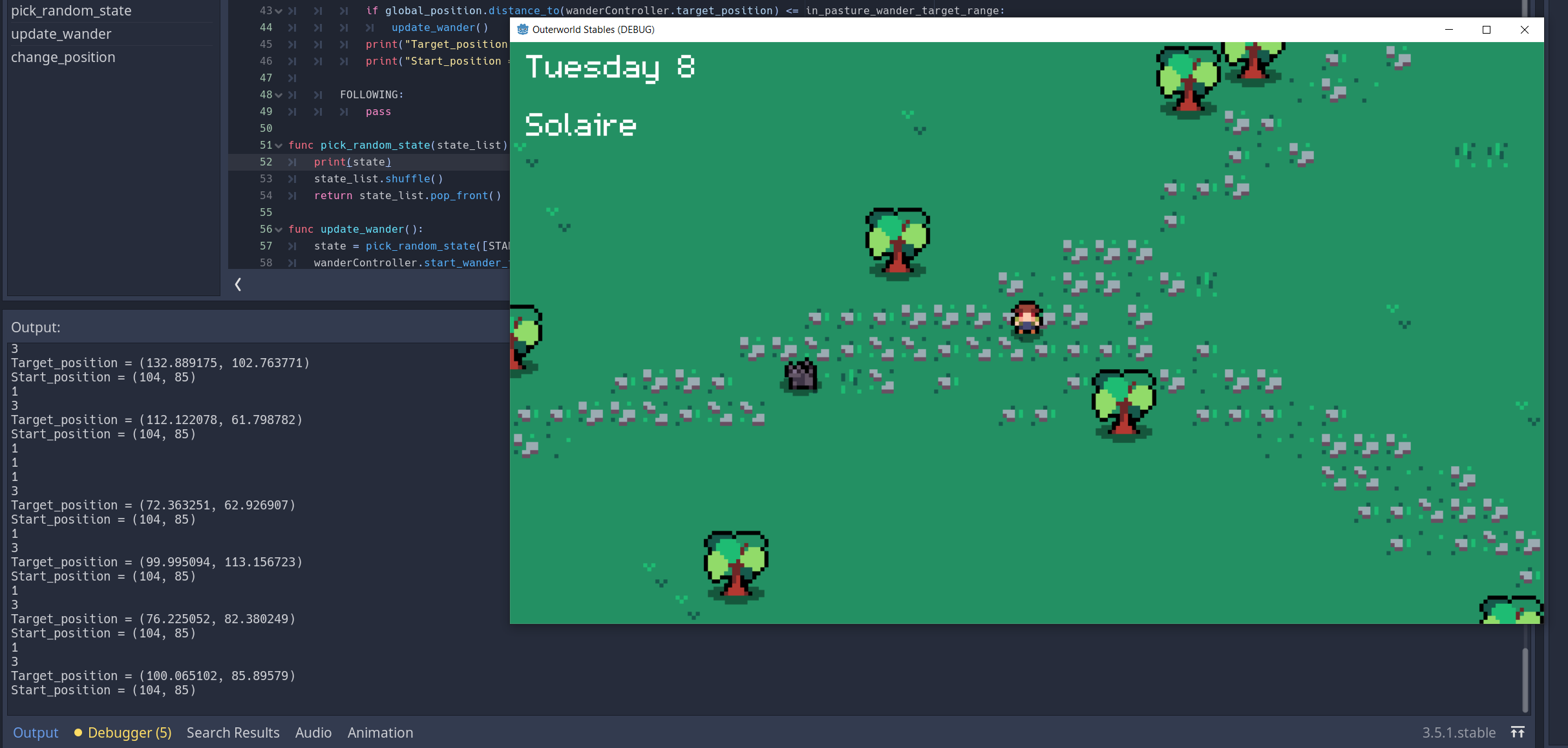
Here's my code:
var wander_target_range = 2
var in_pasture_wander_target_range = 100
export var horse_move_speed = 70
var velocity = Vector2.ZERO
var state = STANDING
enum {
STABLED,
STANDING,
WANDERING,
IN_PASTURE,
FOLLOWING
}
func _ready():
state = pick_random_state([STANDING, IN_PASTURE])
func _physics_process(delta):
match state:
STABLED:
pass
STANDING:
velocity = velocity.move_toward(Vector2.ZERO, horse_move_speed)
if wanderController.get_time_left() == 0:
update_wander()
WANDERING:
pass
IN_PASTURE:
if wanderController.get_time_left() == 0:
update_wander()
change_position(wanderController.target_position, delta)
if global_position.distance_to(wanderController.target_position) <= in_pasture_wander_target_range:
update_wander()
print("Target_position = ", wanderController.target_position)
print("Start_position = ", wanderController.start_position)
FOLLOWING:
pass
func pick_random_state(state_list):
print(state)
state_list.shuffle()
return state_list.pop_front()
func update_wander():
state = pick_random_state([STANDING, IN_PASTURE])
wanderController.start_wander_timer(rand_range(1, 3))
func change_position(point, delta):
var direction = global_position.direction_to(point)
velocity = velocity.move_toward(direction, horse_move_speed)
sprite.flip_h = velocity.x < 0
And here's the code in WanderController:
export var wander_range = 32
onready var start_position = global_position
onready var target_position = global_position
onready var timer = $Timer
func _ready():
update_target_position()
func update_target_position():
var target_vector = Vector2(rand_range(-wander_range, wander_range), rand_range(-wander_range, wander_range))
target_position = start_position + target_vector
func get_time_left():
return timer.time_left
func start_wander_timer(duration):
timer.start(duration)
func _on_Timer_timeout():
update_target_position()
I've set in_pasture_wander_range both high and low and did the same with horse_move_speed, neither seemed to have an impact.