Hi all. Is there a way to determine if a file is of a certain resource type besides just loading it?
I've implemented an asset browser and I want it only to show SpatialMaterial
s. I tried ResourceLoader.exists(path, type)
, but the type doesn't really factor into the method's result. I know I could require users to give materials the .material
extension, but I'd like a more elegant solution, if there is any.
Thanks in advance.
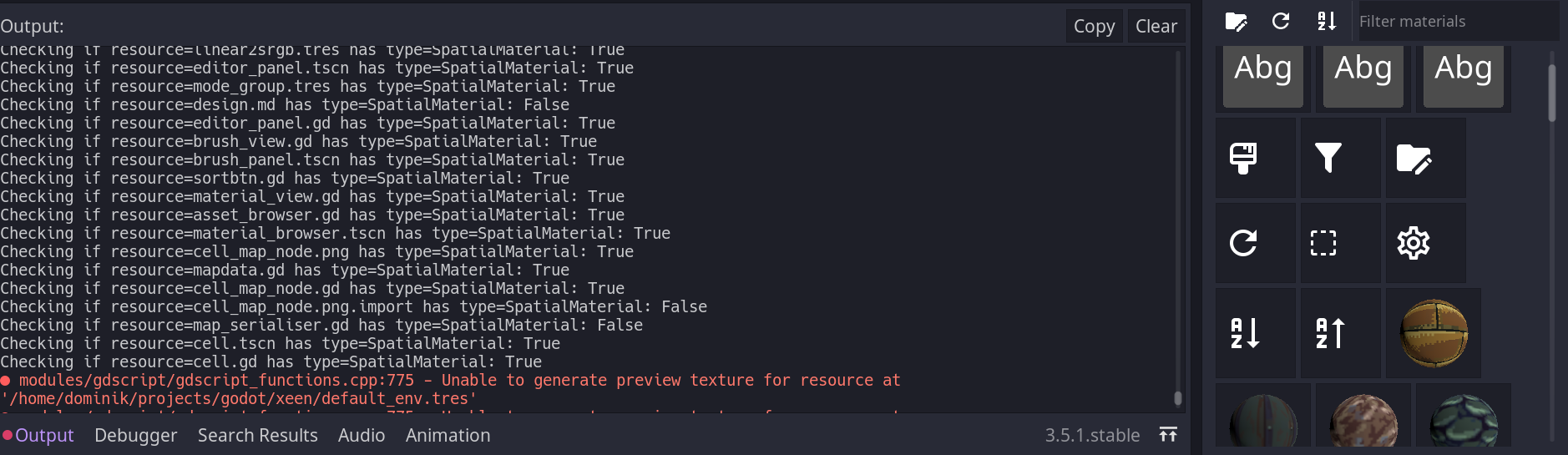
static func _iter_over_files(path: String, f: FuncRef, resource_type: String=""):
var todo := [path]
# Iterate over the given path (as directory) and any sub-directories that
# may be found.
while not todo.empty():
var curr_dir = todo.pop_back()
var directory = Directory.new()
if directory.open(curr_dir) == OK:
directory.list_dir_begin(true)
var item = directory.get_next()
# iterate over items in current directory:
while item != "":
var item_path = _join_paths(curr_dir, item)
if directory.current_is_dir():
todo.push_back(item_path)
else:
var correct_type = resource_type == "" or ResourceLoader.exists(item_path, resource_type) # that's not how you do it...
print("Checking if resource=%s has type=%s: %s" % [item, resource_type, str(correct_type)])
if correct_type:
f.call_func(item_path)
item = directory.get_next()
else:
push_error("Unable to access directory '%s'" % curr_dir)