i'm new to godot. im creating an angry birds type game where you sling a projectile around using drag and release mechanics. I followed a youtube video to create a "vectorCreator" scene that takes mouse or touch input and creates a vector, then impulses the projectile with the created vector based on how far you dragged from the origin of your drag. Anyways, I want to create a sort - of aiming feature. Ideally, when the user pulls back to charge up their drag, I want to display an arc or (ideally a parabola) that follows a path of where the projectile will be flung. This way, it's easier for users to estimate how far or hard they need to pull back in order to reach their targets. I will include the script from the VectorCreator scene and the projectile scene. (the projectile isn't its own scene actually, i haven't abstracted it away. idk if this is an issue but worth mentioning.)
VectorCreator Script below
signal vector_created(vector)
// this var is used as a limit to strength of fling
export var maximum_length := 500
var touch_down := false
var position_start := Vector2.ZERO
var position_end := Vector2.ZERO
onready var center_position = get_node("../projeggtile/CenterPos").global_position
// what we will emit on signal
var vector := Vector2.ZERO
// makes sure our values are reset between flings
func _reset() -> void:
position_start = Vector2.ZERO
position_end = Vector2.ZERO
vector = Vector2.ZERO
update()
func _ready():
connect("_input_event", self, "_on_input_event")
func _draw() -> void:
//draw_line(position_start - global_position,
//(position_end - global_position),
//Color.blue, # blue line the length of the differe
//8)
draw_line(position_start - global_position,
position_start - global_position + vector,
Color.red,
16)
func _input(event) -> void:
if not touch_down:
return
if event.is_action_released("ui_touch"):
touch_down = false
emit_signal("vector_created", vector)
_reset()
if event is InputEventMouseMotion:
position_end = event.position
vector = -(position_end - position_start).limit_length(maximum_length)
update()
func _input_event(_viewport, event, _shape_idx) -> void:
if event.is_action_pressed("ui_touch"):
touch_down = true
position_start = event.position
// Called every frame. 'delta' is the elapsed time since the previous frame.
//func _process(delta):
// pass
Projectile Script below
extends RigidBody2D
#Called when the node enters the scene tree for the first time.
func _ready():
pass
func launch(force: Vector2) -> void:
apply_impulse(Vector2.ZERO, force)
also, here's a screenshot of my root node structure.
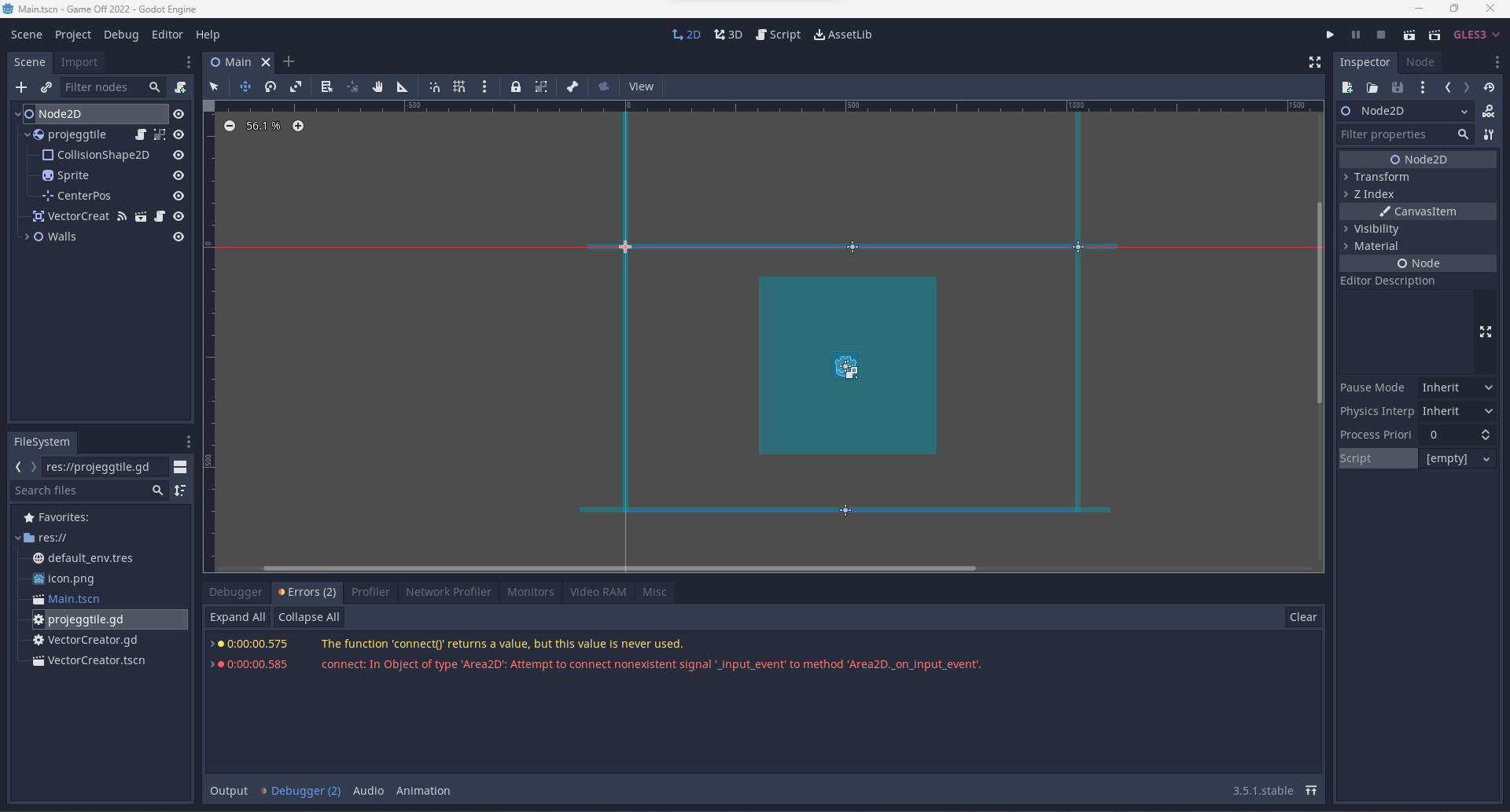
any pointers in the right direction would be awesome!!